Introduction to AWS and Python
Amazon Web Services (AWS) is a cloud computing platform provided by Amazon. It offers a wide range of services with a pay-as-you-go pricing model over the internet. These services include storage, computing power, databases, machine learning, and more. Python, on the other hand, is a versatile programming language that is widely used in web applications, software development, data science, and machine learning (ML). Let’s see how Python and AWS come together:
1. Understanding AWS Service Categories:
AWS services fall into different categories:
- Compute services: These provide virtual servers (EC2), serverless computing (Lambda), and batch processing (Batch).
- Storage services: Amazon S3 for scalable storage, EBS for block storage, and Glacier for archival storage.
- Database Services: RDS for relational databases, DynamoDB for NoSQL, and Redshift for data warehousing.
- Networking services: VPC for network isolation, Route 53 for DNS, and Direct Connect for dedicated network connections.
- Machine Learning Services: SageMaker for ML model training, Rekognition for image analysis, and Comprehend for natural language processing.
- Security and identity services: IAM for access management, KMS for encryption, and Shield for DDoS protection.
- Analytics Services: Athena for querying data, EMR for big data processing, and QuickSight for business intelligence.
2. Services matching application types:
Consider the needs of your project and choose services accordingly:
- Web applications: Use EC2 for scalable computing, S3 for static content, and RDS/DynamoDB for databases.
- Serverless applications: Opt for Lambda, API Gateway, and DynamoDB.
- Data Processing: EMR for big data, Glue for ETL, and Redshift for data warehousing.
- Machine Learning: SageMaker, Recognition and Understanding.
- IoT Applications: IoT Core, Greengrass, and Kinesis.
3. Cost vs Performance Considerations:
Balance your budget constraints with functional demands:
- EC2 Instances: Choose the right instance type based on performance requirements.
- Storage: S3 for scalable storage, EBS for block storage, and Glacier for archival data.
- Database: RDS for relational databases, DynamoDB for NoSQL, and Redshift for analytics.
Setting up your AWS account
To get started with AWS, follow these steps:
1. Sign up for an AWS account:
- Go to the AWS sign-up page.
- Enter your account information (email, company/personal details).
- Read and accept the AWS Customer Agreement.
- Verify your payment information.
- AWS will send a confirmation email when your account is ready to use.
2. Choose an AWS Support Plan:
- Choose a support plan based on your needs (Basic, Developer, Business, or Enterprise).
- Each plan offers different levels of support and features.
3. Activate your account:
- Activation usually takes a few minutes but sometimes takes up to 24 hours.
- Once activated, you will receive a confirmation email.
- You now have full access to all AWS services.
Choosing the Right AWS Services for Python
When working with Python on AWS, consider the following services:

1. Amazon EC2 (Elastic Compute Cloud):
- Launch a virtual server with EC2 instances.
- Use Python scripts to manage and interact with EC2 instances.
2. Amazon S3 (Simple Storage Service):
- Scalable storage for files, images, and backups.
- Interact with S3 using Python and Boto3.
3. Amazon RDS (Relational Database Service):
- Managed relational databases (MySQL, PostgreSQL, etc.).
- Connect to RDS using a Python library like psycopg2.
4. AWS Lambda:
- Serverless computing service.
- Write Python functions and trigger them based on events.
Deploying Python Applications on EC2 Instances
Amazon provides virtual servers in the EC2 (Elastic Compute Cloud) cloud. Here’s a step-by-step guide to deploying Python applications on EC2:

1. Launch the EC2 instance:
- Choose an Amazon Machine Image (AMI) based on your needs (for example, Ubuntu, Amazon Linux).
- Select an instance type (for example, t2.micro) based on the load of your application.
- Configure security groups to allow required ports (e.g., SSH, HTTP).
2. Connect to EC2 instance:
- Use key pairs for authentication (.pem for Ubuntu, .ppk for Windows).
- Enable “Auto-assign public IP” during instance setup.
3. Update packages and install Git:
- Connect to the instance using EC2 Instance Connect.
- Update existing packages: sudo apt update && sudo apt upgrade.
- Install git: sudo apt install git.
4. Clone your Python project:
- Clone your project repository using Git: git clone <repository_url>.
5. Install the dependencies and run your Python application:
- Ensure availability of Python 3: Python3 –version.
- Install project dependencies using pip install -r require.txt.
- Run your Python application (for example, a Flask web app) on a specific port (for example, 8501).
6. Keep the app running:
- Use the nohup command to keep the application from running permanently.
- Refresh the page to see your running app.
- Remember to close the terminal after running the command to keep the application active.
7. Terminate EC2 instance after usage:
- To avoid charges, terminate your work when you’re done.
Serverless Python with AWS Lambda
AWS Lambda allows you to create and configure functions that run in an AWS environment. Here’s how to build a serverless REST API using Python and AWS Lambda:
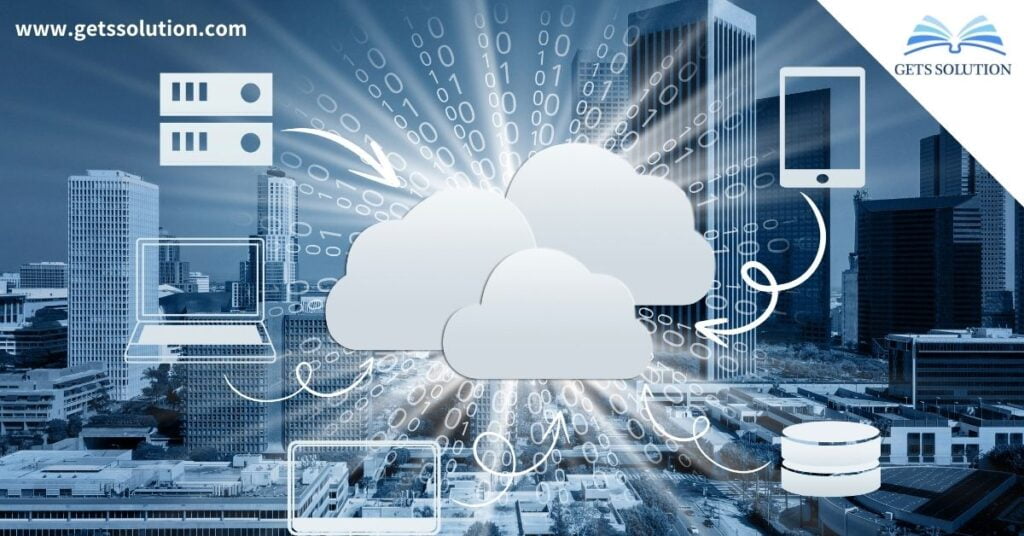
1. Create a lambda function:
- Define a handler function that accepts JSON input and returns it.
- Specify the Python runtime environment (for example, Python 3.8).
2. Define triggers:
- Set up triggers (for example, API Gateway, S3 events) to fire your Lambda function.
- Create IAM roles to allow Lambda to access other AWS services (e.g., DynamoDB).
3. Implement your REST API:
- Create endpoints (for example, POST for submitting data, GET for retrieval).
- Use DynamoDB to store data between requests.
Storing Data: S3 and RDS
Amazon S3 (Simple Storage Service) and RDS (Relational Database Service) serve different purposes:

1. Amazon S3:
- Object storage for virtually any type of data.
- Pay for the storage you use.
- Highly granular access control.
- Ideal for scalable, schema-less storage.
2. Amazon RDS:
- Managed relational databases (MySQL, PostgreSQL, etc.).
- Pay-as-you-go model.
- Supports transactions.
- Suitable for structured data with a specific schema.
Scaling Python Applications
What is scaling?
Scalability refers to an application’s ability to handle increased workloads, adapt to growth, and adjust to changing requirements. When building scalable Python applications, consider the following approaches:

1. Multithreading:
- Use multiple threads in the same process to run code in parallel.
- Be aware of Python’s Global Interpreter Lock (GIL) limitations.
- Multithreading is suitable for CPU-bound tasks when multiple CPUs are available.
2. Multiprocessing:
- Use separate processes (each with its own memory space) to achieve true parallelism.
- Overcome the limitations of the GIL by running Python code in separate processes.
- Multiprocessing is ideal for I/O-bound tasks and CPU-bound tasks on multi-core systems.
3. Distributed Systems:
- Distribute the workload across multiple hosts or nodes.
- Achieve horizontal scalability by adding more nodes.
- Increase fault tolerance by allowing other nodes to take over traffic if one fails.
4. AWS Auto Scaling:
- Take advantage of AWS Auto Scaling to dynamically adjust the number of EC2 instances based on demand.
- Set up a load balancer to distribute traffic evenly.
5. Serverless Architecture:
- Use AWS Lambda for serverless Python functions.
- Lambda automatically scales based on incoming requests.
- Ideal for event-driven workloads.
Monitoring and Logging
Log Monitoring:
- What are logs?
- Logs are records of events that occur within a system or application.
- They include timestamps and event details.
- Types of logs: Application log, system log, security log, etc.
- Log Monitoring:
- Continually monitor logs to identify specific events or patterns.
- Aggregate log entries in one centralized location.
- Standardize log formats for uniformity.
- Evaluate incoming logs in real-time and issue alerts for specific events.
Monitoring:
- Real-Time Visibility:
- Monitor system performance, resource usage, network traffic, and error rates.
- Use monitoring tools to detect anomalies and proactively prevent problems.
- Equipment and Services:
- AWS Cloudwatch, Prometheus, Grafana, Nagios, etc.
- Monitor infrastructure, applications, and services.
Security best practices
- Implement a strong cyber security strategy:
- Conduct a cybersecurity audit to assess your current situation.
- Develop plans and procedures tailored to your organization.
- Update and enforce security policies:
- Review and update security policies regularly.
- Ensure compliance with industry standards.
- Use strong passwords and multi-factor authentication (MFA):
- Enforce password complexity.
- Enable MFA for added security.
- Collaborate with IT departments:
- Work closely with IT teams to prevent attacks.
- Stay informed about current cyber trends.
- Perform regular cyber security audits:
- Evaluate weaknesses and address them immediately.
- Control access to sensitive information:
- Limit access to important data.
- Enforce role-based access controls.
Cost Optimization Strategies
Managing costs on AWS is important. Let’s explore strategies to optimize expenses while maintaining performance:
- Understanding AWS Pricing:
- Pay as you go: Pay for actual usage without upfront commitments.
- Free Tier: Use limited resources for free.
- On-demand example: Pay by the hour or second.
- Reserved Instances (RI): Commit to specific instance types for 1 or 3 years (significant savings).
- Spot example: bid for unused capacity (risky but cheap).
- Reserved Instance (RI):
- Standard RI: Pay upfront, and commit to one instance type (ideal for static workloads).
- Variable RI: Flexibility to change instance types within the same family.
- Scheduled RI: Instances reserved for specific time windows (for example, daily batch jobs).
- Spot example:
- Cheap but unstable.
- Use for fault-tolerant workloads or batch processing.
- Be prepared for sudden termination if demand increases.
- Auto Scaling and Load Balancers:
- Efficient scaling keeps costs under control.
- Set up an auto-scaling group to adjust the number of instances based on demand.
- Load balancers distribute traffic evenly.
- Architect for cost efficiency:
- Multi-AZ RDS: Consider high availability but consider the cost.
- S3 Lifecycle Policies: Automatically convert objects to cheaper storage classes.
- Avoid overprovisioning: Monitor resources and adjust as needed.
- Spotlight on Lambda:
- Cold Start: Optimize by keeping tasks warm or using provisioned concurrency.
- Memory allocation: Fine-tune memory settings.
Conclusion
Congratulations! You are now ready to run Python applications on AWS. Whether building an MVP or handling enterprise workloads, AWS and Python are your dynamic duo. So go ahead, deploy, scale, and conquer the cloud!
FAQs
Q: Is using AWS free?
A: AWS offers a free tier with limited resources. Furthermore, the cost depends on the usage. Be careful what you are doing!
Q: Can I use Python 2 on AWS?
A: Python 2 has reached the end of its life. Stick to Python 3 for security and compatibility.
Q: How do I troubleshoot lambda cold start?
A: Consider provisioned concurrency, optimize your code, and monitor execution time.
Q: What is the best way to estimate costs before deployment?
A: Use the AWS pricing calculator and review your architecture carefully.
Q: Is there a limit to how many bananas I can buy with my AWS savings?
A: Well, that’s a fruitful question! But seriously, focus on optimizing your cloud costs instead.
This gateway is incredible. The splendid substance displays the manager’s commitment. I’m overwhelmed and envision more such mind blowing substance.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
No problem at all! I understand that sometimes even after reading something, there can be things that aren’t entirely clear.
To better understand what you’re unsure about, could you tell me a bit more about the specific parts of the article that left you with doubts? Knowing what areas you’d like clarification on will help me tailor my explanation and hopefully clear things up!
Your point of view caught my eye and was very interesting. Thanks. I have a question for you.
Thanks for letting me know! What specifically were you curious about?